This video is available to students only
Creating Action in Redux
In this lesson, we're going create actions
Project Source Code
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Unlock This Course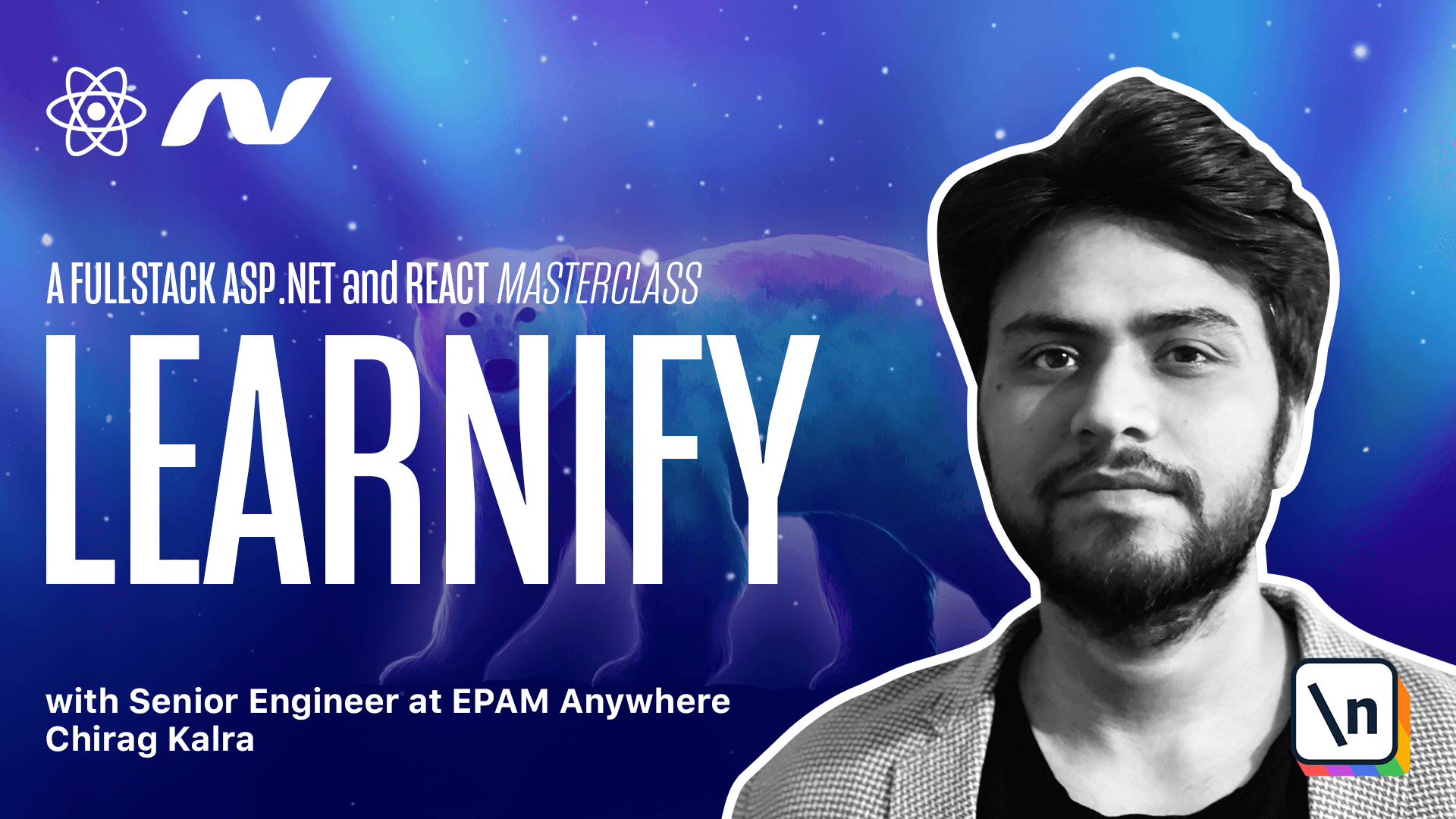
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
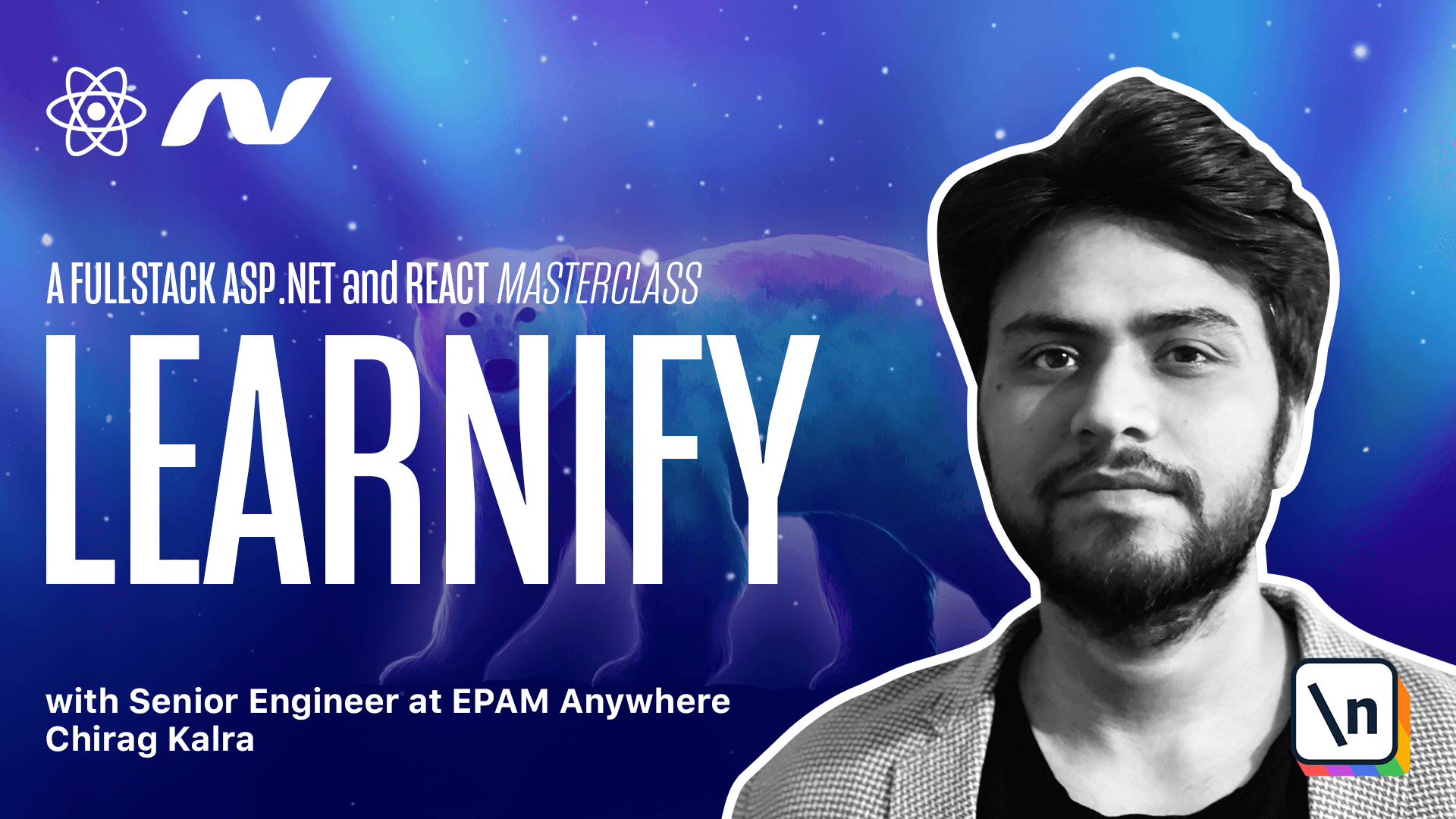