Lectures Controller
In this lesson, we're going to create lectures controller
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
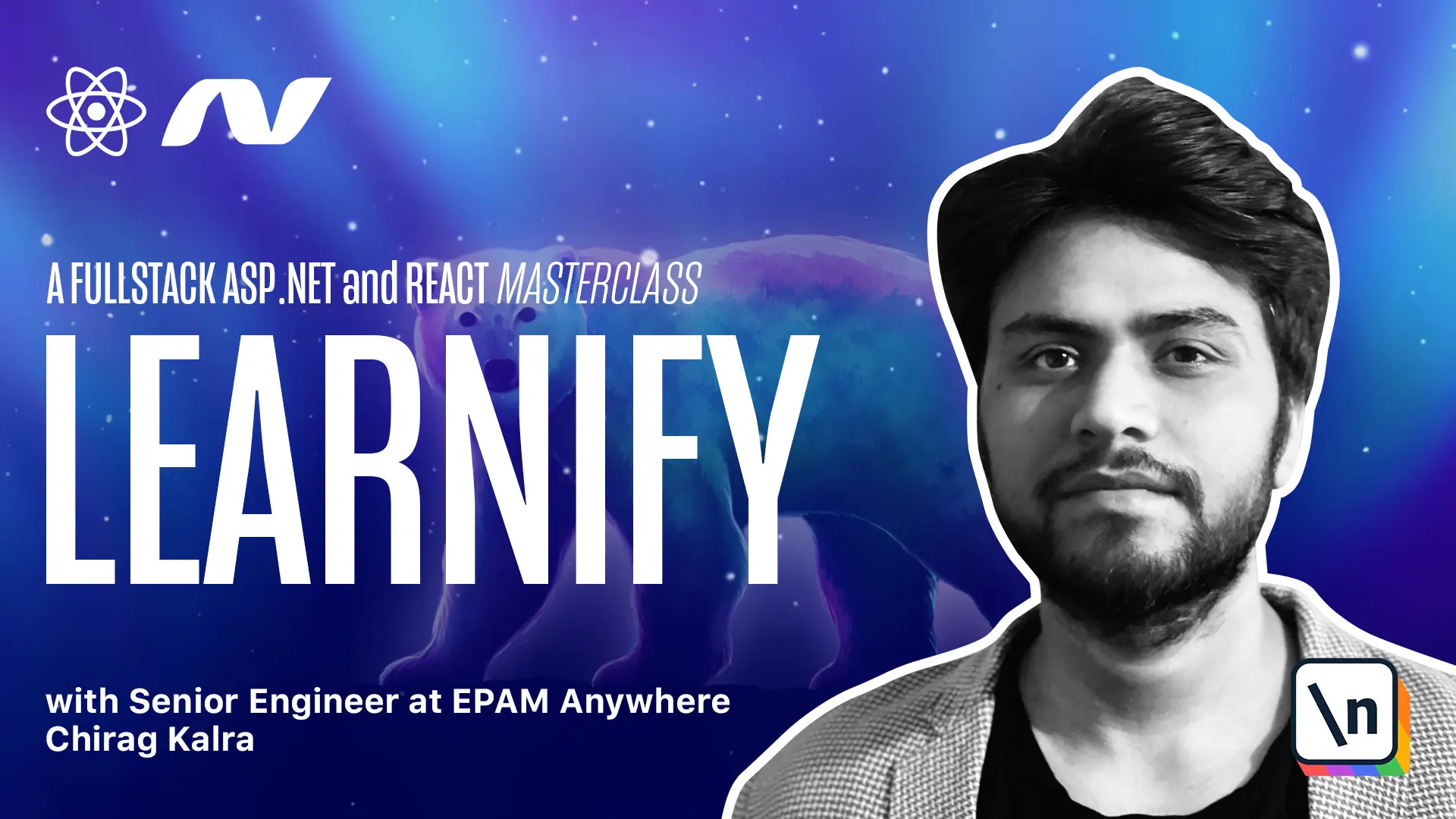
[00:00 - 00:18] Now that we have created section stable and lecture stable with the data, it's time to work on the controller which will send the courses data which include sections and the lectures. Before we start working on the controller, there is one more thing I would like to add to the user cost table, which is the current lecture property.
[00:19 - 00:40] Usually if you take a course, you will notice that it will resume the course where you last left it. What we can do is we can go to the user cost and add current lecture property.
[00:41 - 01:11] This will be of type integer because lecture ID is type integer. Since we have added a new property, we will need to make a new migration so we can open our terminal and close it, go back to the root and let's clear it and now we can run .net ef migrations add and let's call it current lecture added.
[01:12 - 01:21] Our starter project is API and the project is infrastructure. Now we can restart our server.
[01:22 - 01:30] So let's go back to the API project and run .net watch run. Now we can check the user cost table.
[01:31 - 01:40] Let's open our database and we will find the user cost table. This one user cost is stable.
[01:41 - 01:48] Now we see that the current lecture has by default added zero as the initial value. We can now start working on the lectures controller.
[01:49 - 02:00] So inside API and controllers, let's create a new class. This will be called lectures controller.
[02:01 - 02:14] We can close user cost and the terminal for now and as usual, we will derive it from the base controller. And now we can create a constructor and here we will need the store context.
[02:15 - 02:22] So let me write store context with name context. We will need imapper.
[02:23 - 02:31] So that's import imapper with name mapper. And finally, we will need a user manager as well.
[02:32 - 02:41] So let's write user manager with name user manager. Let's import all of them one by one.
[02:42 - 02:52] So we will import store context using infrastructure. Imapper using imapper and user manager using ASP net core identity.
[02:53 - 03:02] And the user manager should be of type user. So let's write user here and let's import user using entity.
[03:03 - 03:09] And now we can initialize the field from parameter. So let's do that one by one for all of them.
[03:10 - 03:20] Let's do that for the mapper and once for user manager. Now let's start working with the get request.
[03:21 - 03:33] So below I can write HTTP get and this request will send all the sections and the lectures back to the client. In this request, we will need course ID from the client.
[03:34 - 03:49] So what we can do is inside brackets, we can mention quotations and curly brackets, which will have the course ID. By doing this, we are expecting the course ID inside the query parameters.
[03:50 - 04:01] Also this request should be authorized because we don't want people who are not logged in to receive courses. So we can write authorize and now we can start writing our method.
[04:02 - 04:22] And now we can write public async task of type action result as usual. And from this function, we want to return the course name list of all the sections and the current lecture.
[04:23 - 04:28] Let's create a new DTO with name user lecture DTO. So let's start with writing it here.
[04:29 - 04:37] Let me write user lecture DTO. Since we don't have it right now, we can create it inside the DTO folder.
[04:38 - 04:48] So inside DTO, let's create a new class and let's call it user lecture DTO. Like I said, we will return the course name.
[04:49 - 05:01] So let's write type string and course name. We want to return the current lecture, which will be of type int.
[05:02 - 05:12] Let's write current lecture and also we want to return the list of sections. So what we can do is we can't return section directly.
[05:13 - 05:19] Otherwise it will create a circular dependency and will give us an error. So for the sections, we can create another section DTO.
[05:20 - 05:36] So here, let me write list of section DTO. So now we can import list using system collections generic and we can create section DTO in a new file.
[05:37 - 05:42] So inside the section DTO, we will have the name of the section. So the name will be type string.
[05:43 - 05:57] Let's call it section name. Also we want to return the list of lectures and like section DTO, we will create lecture DTO so that it doesn't give us any circular dependency error.
[05:58 - 06:09] So let me write list of lecture DTO. Let's call it lectures.
[06:10 - 06:22] Let's import list using system collection generic and let's create lecture DTO in a new file. So our lecture DTO will have an ID because that will be a part of our URL.
[06:23 - 06:42] So let me write ID of type int. We want title which will have a type string and URL of type string.
[06:43 - 06:55] We are returning the URL because this will be responsible for showing different videos. So now that we have created our DTOs, we can map them inside the mapping profiles file.
[06:56 - 07:15] So let's go to mapping profile and let's start with creating a map between lecture and lecture DTO. So let me write create map between the lecture and lecture DTO and another one for section and section DTO.
[07:16 - 07:34] So let's copy it and paste it and replace lecture with section and here section DTO. And here we will have to map a property which is called section name.
[07:35 - 07:57] So I can write dot for member and the property is section name and we will map it from section name. So C dot name.
[07:58 - 08:14] Now that our mapping is finished, let's go back to the lectures controller and let's name this method get lectures. Since we are expecting course ID, so let's write GUID and course ID.
[08:15 - 08:31] Let's make the C small also let's import GUID using system and before writing, let's import task using system threading tasks. We need action result.
[08:32 - 08:43] We need user lecture DTO using API DTO and also import authorize using Microsoft ASP net core authorization. So here we will start with the course.
[08:44 - 09:00] So let's write course which is equal to await context dot courses dot find async and here we will pass the course ID. We also need user ID.
[09:01 - 09:47] So let's make a new variable for the user which will be equal to await user manager dot find by name async and inside we will pass user dot identity dot name because it's an authorized request and we know we will receive the token so we can use user identity name here and now let's create a sections variable. So here let's create sections which will be equal to await context dot sections dot where we want to find the section where course ID of the section is equal to this course ID.
[09:48 - 10:07] So what we can write is X which will go to X dot course ID if it is equal to course dot ID we can import where using system link. So we are only looking for the section which has this particular courses ID.
[10:08 - 10:12] Now we can also include the lectures. So let me write dot include.
[10:13 - 10:26] So let's write see which will go to see dot lectures and finally we will make it list. So let's write to list async.
[10:27 - 10:29] We also need to import include. So let's do that.
[10:30 - 10:36] That's important using Microsoft entity framework. Since we also need to return the current lecture from this request.
[10:37 - 10:58] So to get current lecture we will need user cause. So let's write war user cause and equal to context dot user causes dot where we want to find the user cause where user is this particular user and course is this particular cause.
[10:59 - 11:23] So we can write this where statement twice one for the user we can check if X dot user is equal to user and then we can use it again to check if X dot cause is equal to this particular cause and since we want just one result we can write dot first. Finally we can return the new user lecture DTO.
[11:24 - 11:38] So let's write return new user lecture DTO and inside we can pass the cost name . Which is course dot title.
[11:39 - 11:58] We need sections and for sections we will need mapper. So let's write mapper dot map and we are going to map section and section DTO and that too with the list.
[11:59 - 16:44] So what we can do is we can write list of section with list of section DTO and inside we can pass the sections and it says user lecture DTO does not contain a definition for sections so let's go to use a lecture DTO and here it should be sections we should have changed that and now since this is resolved we finally want to return the current lecture so let's write current lecture and this will be equal to user cause dot current lecture and let's import list using system collection generic and that is simply it. Now let's create another endpoint for setting the current lecture and this time it will be a put request so when this function is ending we can write another one and this will be a put request so let me write HTTP put and it will have a name set current lecture so let's write set current lecture this should also be an authorized request so let's write authorized now let's start writing our method so it's public async task of type action result and we don't want to return anything since we are just updating it let's give it a name and let's call it update current lecture for this method we will need a body which will include the course ID and the lecture ID so here let's write from body and let 's call it update lecture dto and let's give it the same name update lecture dto since we don't have that so inside the dto folder let's create another one let's call it update lecture dto and this will have lecture ID which will be of type int and it will have a course ID with type grid and the name will be course ID let's import grid using system and now we can start writing our method for this method as well we will need the user and the user course so we can simply copy it because it's exactly the same we need user we need user course so let's copy them and paste them here and here we don't have course so what we can do is we can write x dot course ID and make it update lecture dto dot course ID now here we want to update the current lecture so what we can do is we can write user course dot current lecture and make it equal to update lecture dto dot lecture ID and now we can do the same stuff we can write result and check if await context dot save changes async is greater than 0 we are simply checking if the result is successful so we can write if result we can return ok otherwise we can return a bad request and it should be bad request and we can use the new API response that we created and inside we can pass status to be 400 and the message can be problem updating the lecture let's import API response using API.error response now that our endpoints are created let's start working on the front end in the next lecture