Creating Remove Item Method
In this lesson, we're going to create removeItem method
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
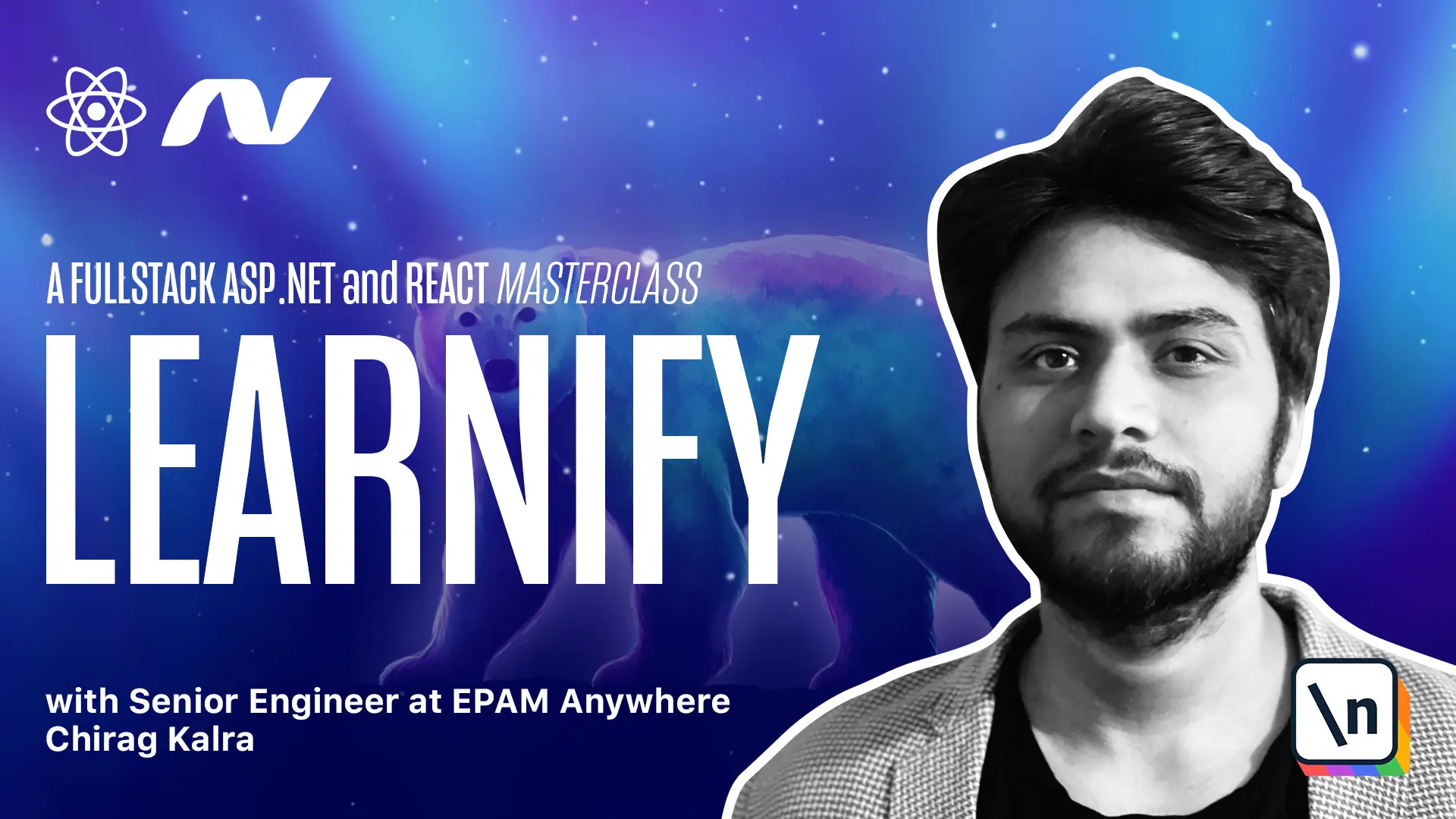
[00:00 - 00:06] Now, we are left with creating the remove item method. So, let's do that right now.
[00:07 - 01:02] Let's do it below the add item to basket method and I will create HTTP delete and below that public async task of action result and let's call it remove basket item and it will again accept cause ID so we can write cause ID and as you can see it's not returning anything because we don't have to when we are deleting an item. First of all, we will get the basket using our extract basket method.
[01:03 - 01:13] We will await extract basket. If the basket is null, we can return the not found response.
[01:14 - 01:44] So, we can write if basket is equal to null return not found and we can use our new API response and it will be 404. We are not going to create a new basket in this case because customer who wants to remove item which means they most probably have a basket already.
[01:45 - 02:02] But if the basket is not null, we want to use the remove cause method which we created and pass the cause ID. So, what we will do is, basket dot remove cause and inside we will pass the cause ID.
[02:03 - 02:21] We can again check if the changes were saved to the database. So, I will create again var result and check if our context dot save changes async is more than zero.
[02:22 - 03:00] If there are changes which means if result, we can simply return okay. If there are no changes made, we can return bad request and again we will pass our new API response with 400 status code and the message can be problem removing the item from the basket.
[03:01 - 03:13] Now, we don't want to send the basket item as it is to the client because like before, it will create a circular dependency. So, what we will do is create our basket dto.
[03:14 - 03:32] What we can do is simply open our basket entity and we can copy the client ID and the items. Now inside API and dto, let's create our new class which will be basket dto.
[03:33 - 03:44] So, we can paste the properties here. We can change the basket item to basket item dto and we don't need this here.
[03:45 - 04:01] Now let's import list using system dot collections dot generic and using quick fix, we will generate class basket item dto in a new file. Now let's think what we want to return to the client.
[04:02 - 04:14] We can return the cost ID which will be of type GUID. Let's import GUID using system.
[04:15 - 04:45] We can also return the title which is of type string. We can return the price which is float image of type URL of type string, sorry image and the instructor as well which is again of type string.
[04:46 - 04:54] This looks enough for now, we can now go to the mapping profiles class and map them together. Let me search for mapping profiles.
[04:55 - 05:07] Let's save the changes. First, let's create a map between basket and basket dto.
[05:08 - 05:22] The next map will be between the basket item and the basket item dto. So, create map.
[05:23 - 05:59] First one is basket item and second one is basket item dto. This time we will use for member, the first one will be the cost ID and we will map from ID which goes to c.cost ID.
[06:00 - 06:12] We can copy it four more times. So I'll copy it and one, two, three, four add a semicolon to the end.
[06:13 - 06:33] Now replace this cost ID with title and here it will be c.cost.title. This time let's do it for the price b.price and here it will be c.cost.price.
[06:34 - 06:57] And for the image b.image and here c.cost.image and finally for the instructor b.instructure and we will map from c.cost.instructure. We can go to a basket controller now.
[06:58 - 07:07] So let's go to the basket controller and inject automapper to our constructor. So we will use iMapper as you know.
[07:08 - 07:24] So iMapper and I will simply call it mapper. Let's use automapper to import it and also initialize field from parameter.
[07:25 - 07:36] However we are using basket we can replace it with basket ddo first indicate basket method. Here let's create a new basket response variable.
[07:37 - 08:15] So var basket response and write mapper.map and here we will map the basket with the target basket ddo and inside i will simply pass the basket. Let's import basket ddo using api.ddo and now we can simply return the basket response instead of basket.
[08:16 - 08:40] We can do the same thing inside our add item to basket method. So what I will do is copy it here and here let's make basket basket ddo and below I can use the same mapper and we can return basket response rather than returning the basket.
[08:41 - 08:48] Now we are ready to check if everything is working fine. Make sure the server is running and open postman.
[08:49 - 09:02] Let's open module 8 and run add cause to basket method. It already has cause id in the query just press send and we see the client id and the cause.
[09:03 - 09:08] So this works well. Let's try and run this again.
[09:09 - 09:17] And this time we see an error which means it's working fine. Let's run the get basket request now.
[09:18 - 09:24] Let me run this. We see the cause we just added.
[09:25 - 09:34] Finally let's run the remove item. So this has the id which we used here.
[09:35 - 09:38] So this is the cause which is in our basket. So now I will remove it.
[09:39 - 09:43] Press send. Now this is successful as well.
[09:44 - 09:52] Press again run the get basket method to see if we have something now and we have nothing. Everything's working as expected.
[09:53 - 10:04] Right now we are using the same API through which we are circulating the cookie . But when we interact with the client and the domain changes we need to add another method to our cause property.
[10:05 - 10:27] So what I'll do is minimize this and let's open our startup class and inside the add cause method I will add dot allow credentials as well. Also we can add order by property to our chain.
[10:28 - 10:47] Let's go to the basket controller and here we can add order by. We can take this and order it by id.
[10:48 - 10:57] Let's import order by using system.link. We are doing this because if we don't do it we will again see the order by warning in our console.
[10:58 - 10:58] And well that's it for now.