Finishing pagination to the API
In this lesson, we're going to finish pagination
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
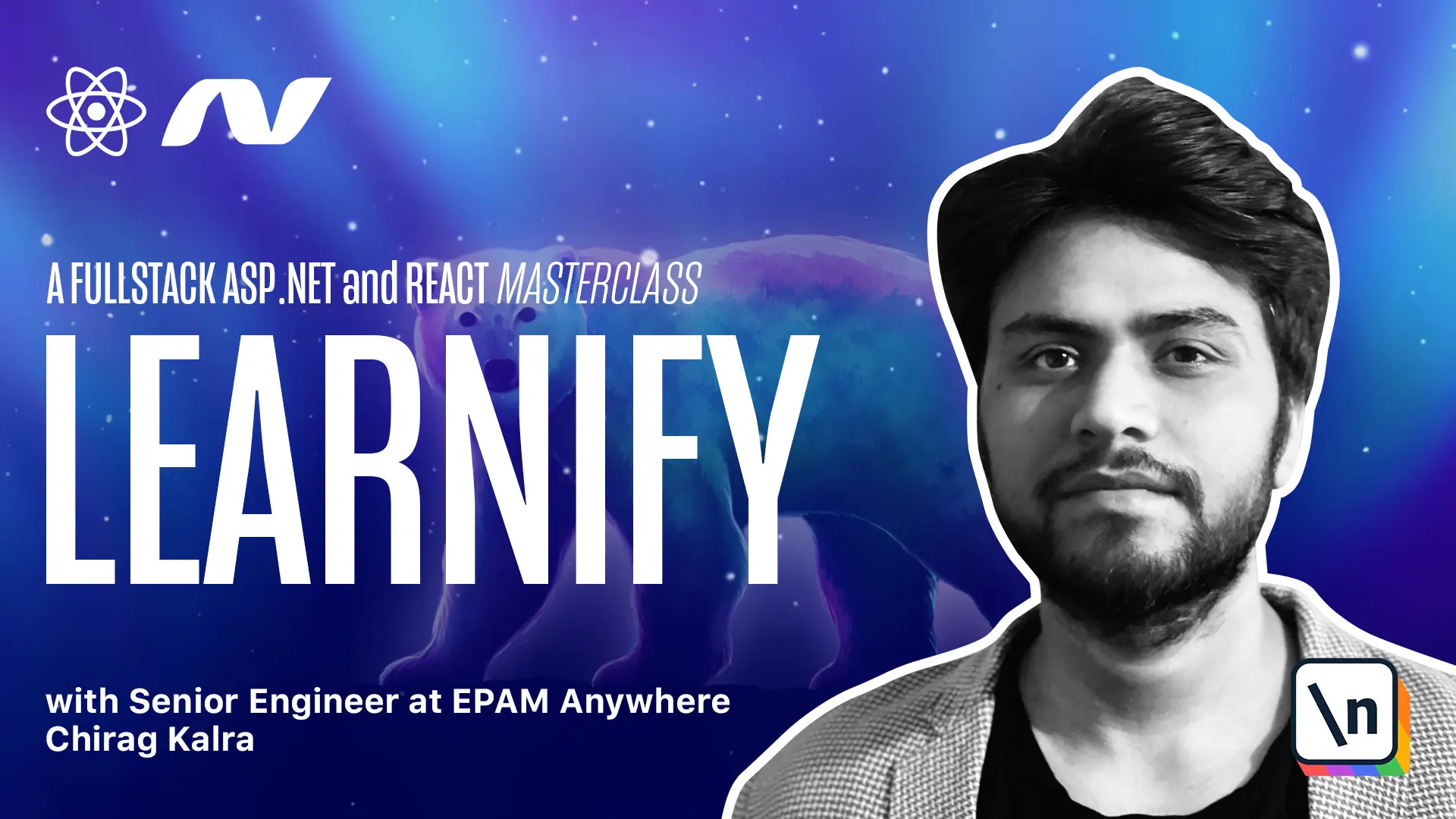
[00:00 - 00:12] Now, we are getting paginated results, but there is some additional information I would like to add to the response. Information such as the total count, the page size and the page index.
[00:13 - 00:27] That will give us more flexibility in the front end I think. So what I'll do is, inside helpers I would create a new class and we'll call it pagination.
[00:28 - 00:36] I will make it generic. So we'll give type t and constrain it with type class.
[00:37 - 00:43] So basically everyone can use it. In the pagination class, I will add four properties.
[00:44 - 00:56] The first one is the page index of type int. So let's call it page index.
[00:57 - 01:10] This will be the page size and it will be an integer again. We need count as well of type int which will have the total count.
[01:11 - 01:42] Finally, we want to return the data which we are already returning and this will be i read only list of type t and we will simply call it data. Let's import it using system collection generics.
[01:43 - 01:54] This type t will be replaced by the actual type which is cause dto in case of courses controller. Now, let's create a constructor using all these properties.
[01:55 - 02:08] We will use this one generate constructor dot dot dot. Talking about the count property, we will create a new specification whose responsibility will be to give us just the count.
[02:09 - 02:21] We want to count the number of results after all the other filters are applied. For example, customer is looking for a cause with rating more than 4.5 and price below $20.
[02:22 - 02:35] We want to first do all this filtering and finally count the result. Although we have created an apply pagination method, but its job is to give us the result by skipping and taking not counting them.
[02:36 - 02:49] So what we will do is create a new method which will count the results for us. For that, we will have to go to our i generic repository since this is going to be generic.
[02:50 - 03:10] Let's create a new method. This will return task of type int because we are going to return the count and let's simply call it count result async.
[03:11 - 03:21] As parameter, it will take a specification of type t again. Now let's implement this inside our generic repository.
[03:22 - 03:37] Let's use implement interface and it has created this method here. We need to pass specification here because we want to count after all the filters are applied.
[03:38 - 04:04] So first of all, we will make it async and here we will return await and we will use the apply spec method and simply use count async method. Now while passing the specifications, there is absolutely no need of passing the include method.
[04:05 - 04:12] Let me open courses with category specification here. You know, we have the include method apply pagination method.
[04:13 - 04:19] So when we are going to count, this doesn't matter. We don't want to have the navigation property.
[04:20 - 04:48] So what we'll do is create a new class inside specification and we will call it courses filters count specification. Again it will derive from the base specification and with type cause.
[04:49 - 05:03] Now let's create a constructor with criteria. In the parameters, we can pass the cause params and we will call it cause params.
[05:04 - 05:20] Inside base, we can simply copy from courses with categories specification just this part. Let's copy it and paste it inside our base.
[05:21 - 05:50] Let's go to our causes controller now and I want it to be clean so I will remove the other tabs. Now inside our get causes method, I will create a new variable called count spec which will be equal to the new causes filters count specification.
[05:51 - 06:03] And it will take cause params as well. To get the total items, we can pass the counts back to our count result async method which is inside our generic repository.
[06:04 - 06:23] So what I'll do is create a new variable total and this will be await repository dot count result async. And we will pass the counts back.
[06:24 - 06:29] If you remember, we don't want to return the I read only list now. We want to return the pagination class.
[06:30 - 06:46] So what we'll do is replace list with pagination. Let's import pagination using API dot helpers.
[06:47 - 07:21] Now we will create a new variable data since data is a property inside pag ination class and this will be equal to this section which we were returning previously. So I will cut it and paste it to the data variable and inside okay, we can create a new pagination class, new pagination of type cost TTO since that's what our list is going to take.
[07:22 - 07:45] Inside we want to return the page index so we can write cause params dot page index, cause params dot page size. We want to send the total and the data.
[07:46 - 07:54] And this is what's required by our pagination class. See we have the page index page size count and the data and that's what we are returning here.
[07:55 - 08:04] Now we can open postman and check if it's working as expected. Let me run this request and here we see the count.
[08:05 - 08:20] We see the page size and the page index. So the page index is two, page size is eight and the count is nine and we are only receiving one if I changed the page index to one.
[08:21 - 08:36] We see the count is still nine but this time we are returning eight results. Now inside the category if I change category to two we see that the count is zero.
[08:37 - 08:40] So that works fine as well. Now let's work on the search filter in the next lesson.