Creating Lecture Page
In this lesson, we're going to create the lecture page
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
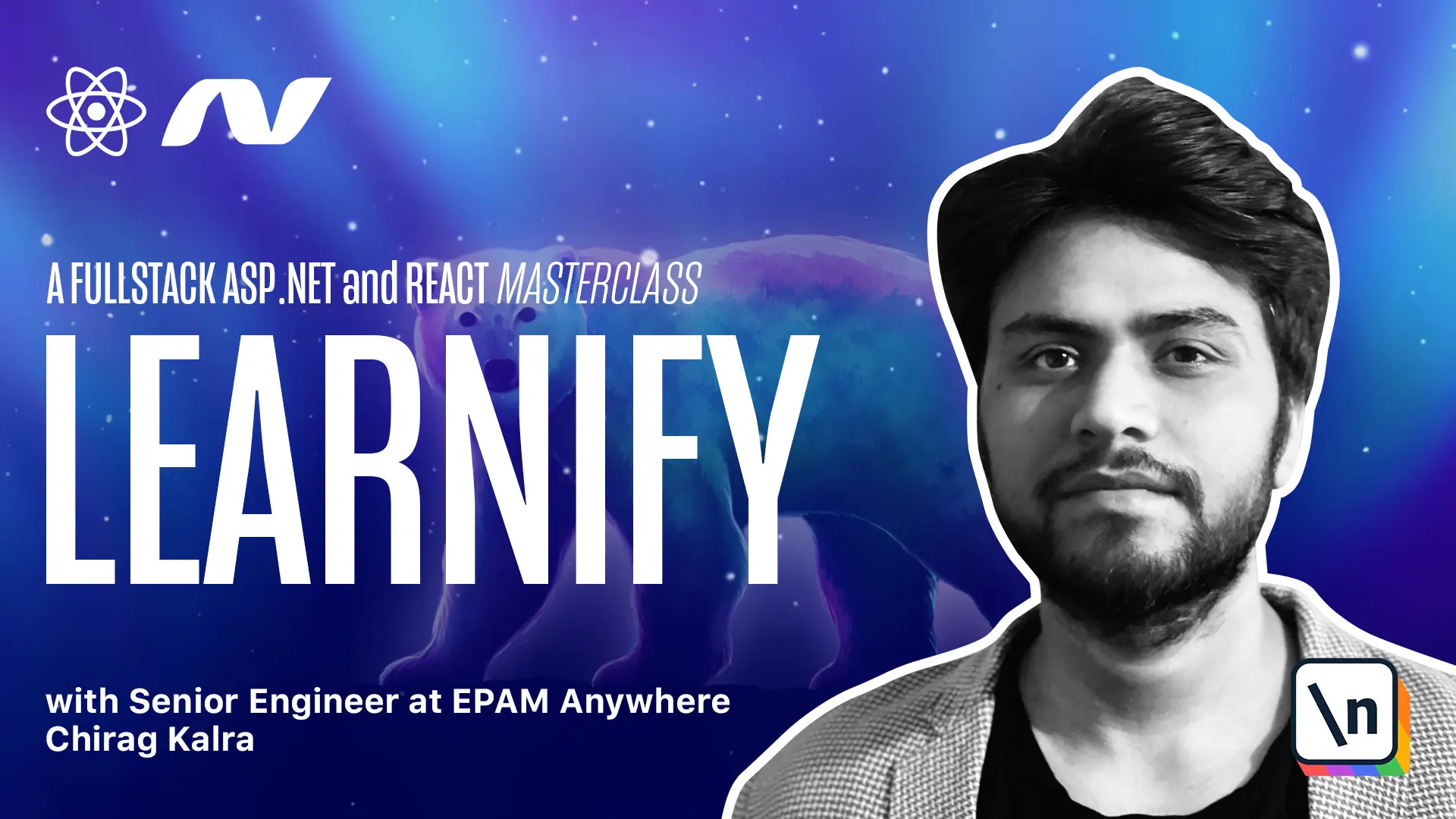
[00:00 - 08:43] Now that we have our endpoints and the lecture slides set up, we can start writing our course page. So let's start clean close everything and Inside pages we can create a new page. Let's call it course page Because here we will display the course Let's start with the function course page And for now let's return h1 which will say course page and Finally we can export default Course page Now we can go to the app.t6 file to register it. It will be a private route as well. So Let's copy one of it and paste it Now let's write the URL of this course page and this will be slash learn Slash course ID Slash lecture ID And since course ID and lecture ID will be dynamic we can add colon in front of them Adding colon means they are dynamic and that can change Let's make it simple and keep it course and Lecture because we know we are talking about the course and the lecture ID Now let's go to the show courses component And we need to wrap go to course with the link So here when we are saying go to course we are pushing the user to the course page so what we can do is we can cut it and use link to wrap it and Paste it back now this will send the user to Since our URL is slash learn so let's write slash learn Slash course so course here can be the course ID so we can write course ID and Slash lecture So let's write slash and for the lecture. We will need current lecture. So what we can do is on top we can use current lecture And import it using use app selector not dispatch from state and state dot lecture And now here we can write slash current lecture So when user clicks on go to course it will send the user to learn slash to that particular course and To the current lecture which can be zero or which can be something else if the user has seen the cost and For now this will work So let's start working on the course page So now we will import lecture lecture loaded current lecture and current video from state so let's write const and import lecture and This will be from use app selector from state and from state it's getting it from state dot lecture and We also need lecture loaded We need current lecture and current video These are the properties. They will need inside this component And we also need dispatch. So what we can do is below this we can write const dispatch Which will be equal to use app dispatch Now let's create a use effect hook and Inside we can check if lecture is null and If it is null we can dispatch they get lectures async call. So let's check if There is no lecture and if it's not there, so we can dispatch Get lectures async and We want to pass the course ID here as a parameter. So let's write course ID And now we want the ID which is inside the URL and React router gives us match as a prop. So here we can de-structure match and the type is route component props and let's give it type any for simplicity and This match has all the properties inside our URL. So our match has params and our params has course If you remember we have written course and lecture as dynamic properties and those properties will be available by using match Dot params dot course And now we can pass dependencies here So let's write dispatch We need match and we need lecture and match and a comma We can create another user effect hook here. We will check if current lecture is zero So let me start writing it and side-by-side. I will explain it to you. So that 's right another user effect hook So inside this user effect hook we will check if current lecture is Zero which means the user has opened the course the very first time. So now we can check if current lecture is zero In this case we can set the first lecture of the first section as the current lecture and first video of the first section can be the first video. So here we can dispatch set current lecture to be lecture dot sections and we can choose the first section and from the first section we can choose the first lecture and We can choose ID of the first lecture and the same way we can set current video To be lecture sections and the first section and from the first section we will choose the first lecture and add that video to be the first one and It should be a URL And now if the current lecture was zero and we don't have any video with lecture zero So what we can check is if there is current lecture which we have set in that case We can push the user to that particular lecture. So for that we need history So what we can do is below dispatch we can write history which is equal to use history and We will check if the current lecture is not zero in that case. We will make history dot replace and Send the user to the current lecture. So what we can do is use Bactics and Inside we can use current lecture. We are doing this because the first time user opens the course The current lecture will be zero. So we are setting the first lecture to be the current lecture and The first video of the first lecture to be the current video. That's what we are doing here And now we are left with one more scenario. We are user refreshes the page We can get the current lecture ID and course from the URL But we really don't know the current video for this we can use the current lecture ID and find its video URL So for that what we can do is we can create a new variable called lecture item So here, let's write let lecture item Which has a type of lecture dto?
[08:44 - 20:54] Let's write lecture dto Now we can loop over the lecture sections for which we will use for off loop So let's write for and inside. Let's write const item of lecture dot sections Lecture item will then be equal to item dot lectures dot find now we want to find the lecture Which has the same ID as this so now let's make it lc Which will check if lc dot ID is equal to the current lecture, which is again an ID since it can be null we can again pass an exclamation mark Now if lecture item is there which means we have found that particular lecture now we will check if the lecture item is not null in this case we can dispatch Set current video to be lecture item dot URL And again we can pass exclamation here And after that we can finally return Now here, let's pass the dependencies which are current lecture dispatch lecture and history Now here what we are doing is we have the lecture, but we don't have the video when the user refreshes the page So what we are doing is with the lecture ID We are going and finding that particular lecture and setting current video to be the URL of that particular lecture And that's it and this will happen only when the user refreshes the page Otherwise when the user will click on that particular lecture It will automatically set current video to be the video of that particular lecture Now let's create another function When user will click on the new lecture in this case we want to send the current lecture ID to the Redux as well as to the database And we want to change the URL to the latest lecture ID So what we can do is after this use effect moving a little up so let's write the function called select lecture and as parameter we need ID of type number and URL of type string And since it's going to be an asynchronous function, let's write async here So we will call this function when the user click on that particular lecture So when the user does that first of all we want to history dot push the user To that particular lecture so to that particular ID After that we want to dispatch set current video, so let's write dispatch Set current video which will be the URL Also we want to make an API call which will set lecture inside the database as well so what we can do is write await and dispatch inside we can call set current lecture async function and as parameter we can pass lecture ID which will be the ID and Course ID which will be match dot params dot course We can now start writing the layout First of all if lectures loaded is false So we will check if lecture loaded it is false In that case we simply want to return the loading component. So let's write loading And that's it and inside the return statement we can first of all Start by creating a dev called lecture And we can divide our lecture into two parts We can keep the lectures sidebar to the left and the video player to the right now Let's start with the sidebar. So here. I will write lecture underscore side bar On top we will have the name of the course. So what we can do is we can create a class lecture Underscontersco sidebar Underscontersco Course this will basically the name of the course. So here we can use lecture dot course name and Here we need to put a curly bracket Now below this we want to loop over the sections and the lectures. So first of all, let's check if lecture is not null So if we have lecture In that case we want to loop over lecture dot sections. So let's go a little down and here Let's loop over the sections. So lecture dot sections dot map and Individual item will be called section and we also need the index. So let's write it here and from here we are going to return and Let's make a div here, which will be the base one and Inside div we will have key Which will have the value of index to make it unique Inside we can create the name of the section. So let's create a new div lecture Underscontersco sidebar Underscontersco section And here we can write the name of the section, which is simply section dot section name Now below this we can loop over all the lectures inside the individual section. So what we can do is Let's create section dot lectures dot map The individual lecture will be called lecture and we will also need an index And from here we will return a link. So let's import link We are using a link here because we will change the URL when we click on that particular lecture and to the link we will pass key, which will be the index And we also want on click event here. So let's write on click And when someone clicks on this lecture, we want to invoke the select lecture function, which takes id Which is the lecture id and URL, which is the URL of the video. So here we can call select lecture function and lecture id will be lecture dot id And lecture URL will be lecture dot URL And we want to highlight the selected lecture. So we can give it a dynamic class name So what we can do is we will write class name and check If lecture id is equal to match params lecture, so let's check lecture dot Id and let's make it string because our URL is always a string And this is equal to match dot params dot lecture And if it is true We want to give it a class name lecture Undiscondisco side bar Undiscondisco list Undiscondisco item Undiscondisco active So if the lecture id and the id inside our URL is same we want to highlight it And if it's not we can just copy it and remove active from here And we see an error because our link needs a two property So what we can do is we can send the user to the lecture id So let's use backtics And here we will simply send the user to lecture dot id Let's save it And now inside the link we will use an icon and the title of the lecture So inside we can create two spans the first one will carry the icon And the second span which will carry the title So here we can pass lecture dot title And for using the icon we will import faeikens So let's go on top And here let's import star as fa icons from react icons slash fa And inside our span we will use faeikens dot fa play icon This one And we also need to give it a styling So let's give it a class name which will be lecture Underskonderskow Underskonderskow icon And this was it for the sidebar And now below this we can write a video player And video player is very straightforward. So let's see where our sidebar is ending So here and below this we will create a video player. So let's give it a class name lecture Underskonderskow video And the video player is going to be very straightforward So we are going to use iFrame So let's start with iFrame And our iFrame will have width of 100% And height of 100% It also needs a title. So let's give it a title of our project which is learnify And the source of the video will be the current video. So let's give it src to be current video For simplicity, I have used youtube videos inside the URL But in a real world application, you would not like your paid videos to be available for free So you can use video hosting services like vimeo or wistia Since I have used youtube's URL, I am using iFrame Now before opening the browser, wrap this code inside an f statement And inside we want to check if we have a lecture Only then we want to execute this And this is just to be safe because we don't want it to run when lecture is null Also, I have provided you a file called lecture.scss That file has all the styles related to this lecture page So what you can do is copy that file go to sass inside pages create a new file Let's call it lecture.scss Paste the code here and finally inside main import it And here it's pages slash lecture And now we can open the browser Make sure you are logged in and you have what?
[20:55 - 21:51] This course full stack python with Django Because only for this course, I have added five sections and five lectures So make sure you buy this course and then open your profile And now you can click on go to course And now as you can see, this is how our lecture page looks like we have sections and for simplicity, I have just called them section one section two section three section four section five And every section has five lectures Like this And also I have included youtube videos So you can click here and you will see URL changing. So if you click on three You will see a new URL and a new video And here we have our course name and below this we have section then we have lectures So this is how our lecture page looks like