Validating the Token
In this lesson, we're going to validate the token inside our server
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
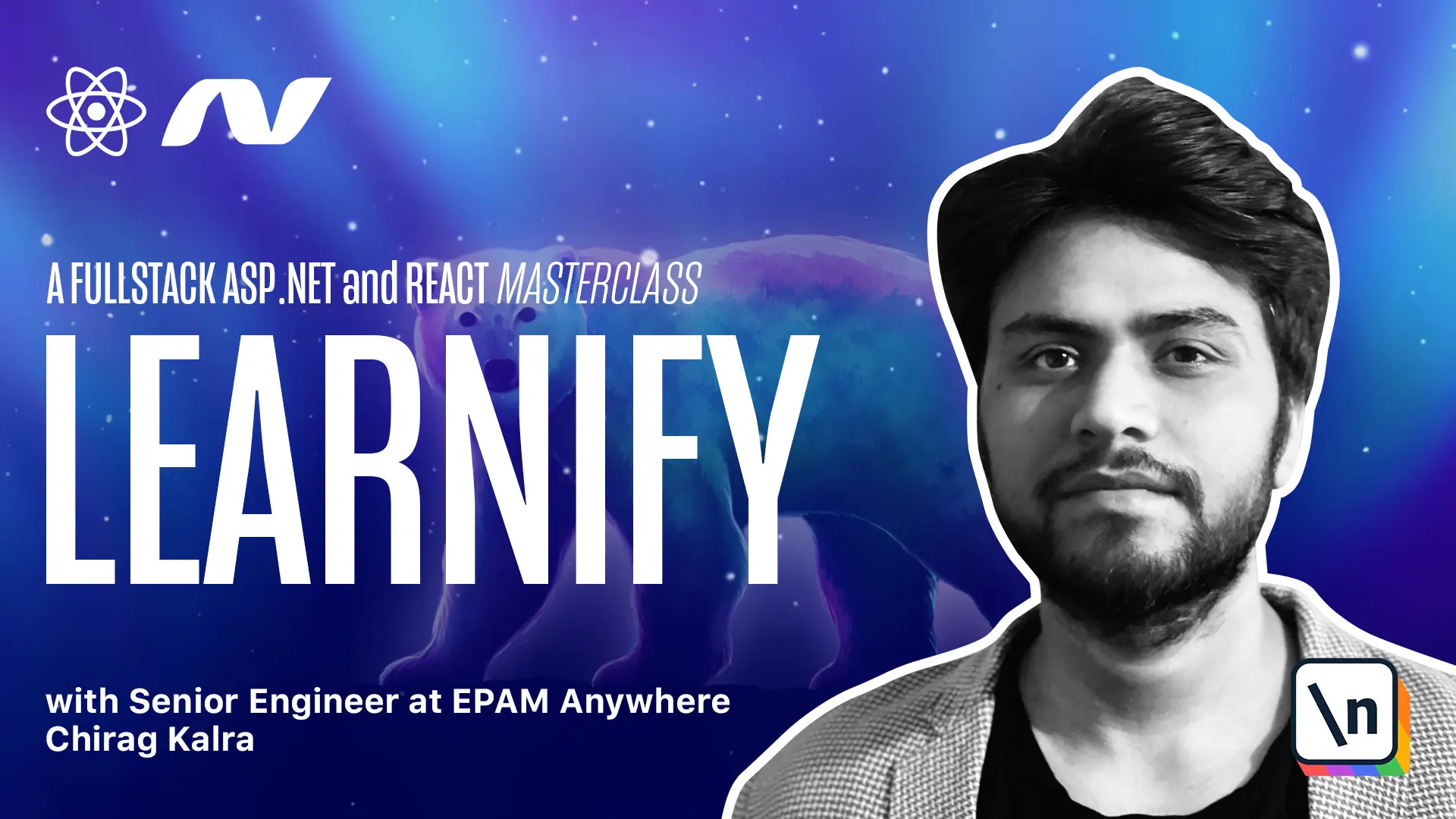
[00:00 - 01:07] Now that we know the token is generated by the server and it has all the properties that we added now we need to verify if we can use the same token to verify the users. To test this we can go to our errors controller. So let's close everything. Let me close the terminal and for time being let's close everything to make it clean. And now let's go to the error controller. Here we can create a new method which will be accessed only if we are authorized. So on top let me make another request and this will be a get request and we can call it auth check. Below this we will add an attribute which will be authorized. So it should be authorize attribute which will only approve the request if the user is authorized. You can also use this authorize attribute on top, on top of this method but that would expect all the requests to be called by the authorized user. But we don't want that.
[01:08 - 01:41] Now let's import authorize from Microsoft ASP netcode.authorization and this is just a method which will return a string. Let's write the method now. So public action result and it will simply return a string. Let's call this method check authorization and it will simply return a string which will say you are authorized.
[01:42 - 03:04] We can now open postman and let's close this. Now we can go to module 6 and run the check authorization request. So if we click on send we see an error. Well what we can do is we can try to log in and here let's log in as a student and let's copy the token going back to this request and since we are using JWT error scheme what we can do is here we can add authorization and write bearer space and then we can pass the token. Now if you click on send we see the same error. Why is it so? Well we have written the code for generating a token but we haven't yet configured it to read the token and verify the user. Now what we can do is go back to the startup class and configure the authentication service. So let's go back and I'm going back to the startup class we need to configure this authentication service.
[03:05 - 03:33] First of all we'll have to add an authentication scheme which will be JWT bearer defaults and the scheme is authentication scheme so I would write authentication scheme. Now we need to import it and you can import it using ASP netco authentication.J WT bearer.
[03:34 - 04:49] After this we have to chain it to add JWT bearer which will take some options so I can write opt and here we need to pass some options and the options are the token validation parameters. So I can write opt.token validation parameters which will be equal to new token validation parameters. Let's import it using Microsoft identity model tokens and inside the curly brackets we'll have to mention the properties. If we see the token service let's go back with the token service we have some properties here and this is basically what we want our token to check upon. So here we have set the issuer and the audience to null. So we'll have to do the same thing here as well. So what I can do is I can write validate issuer to be false.
[04:50 - 06:13] This is nothing but our API which is the local host. Also we can turn the validate audience to be false so let me write validate audience and make it false and our audience is nothing but our client. We don't want to put the constraint on some local host address so we can turn it to false as well. We also have a property called validate lifetime so let's see validate lifetime and since we are checking the expiry of the token we can make it true. And we definitely want to verify the issuer signing key so what we can write is validate issuer signing key and make it true. Now using validate issuer signing key we 'll check the secret token key we have provided for token. Finally we can tell it where to get that key from. So here we will write issuer signing key and make it equal to the key that we have written inside our token service. So what I can do is to show you that it's exactly the same one we can simply copy it and paste it inside our startup class. Now we can import encoding using system.
[06:14 - 06:49] txt and let's put semicolon to get rid of the errors. If we open a service terminal to see everything's working fine and it looks like things are working fine and we are still left with one thing though. Now we just have to add authentication to the middleware. So inside the middleware on top of app.use authorization we have to use app.use authentication. Order is very important here so just make sure that authentication is on top of the authorization.
[06:50 - 07:08] Now this is the configuration part. Now we can go back to postman and let's open body and try to make that request again and see we see you are authorized. Perfect. Now we can start working on the front end in the next lesson.