Using React Context
In this lesson, we're going to implement React context to centralize state
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
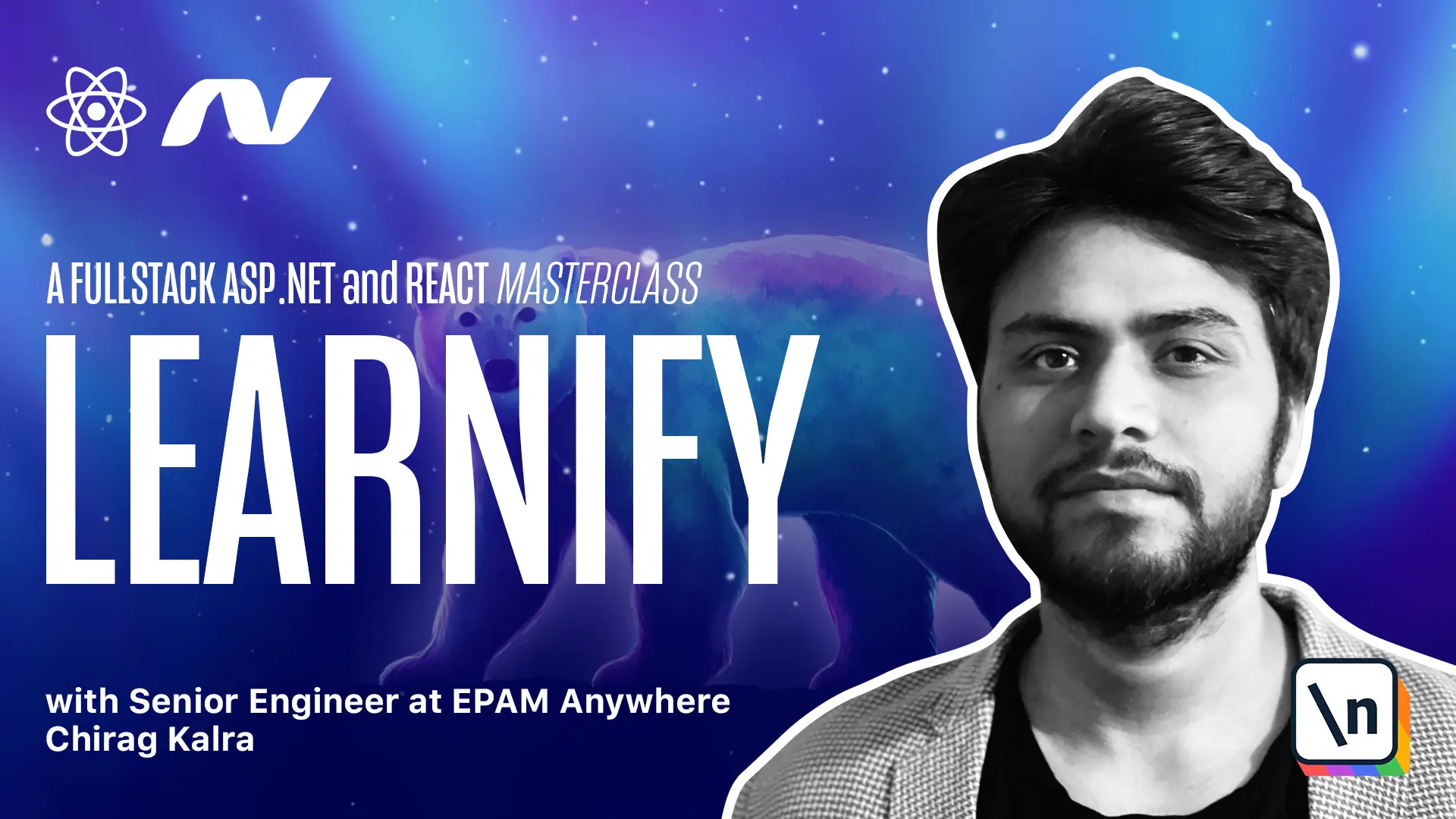
[00:00 - 00:16] Basket is something we want to update from any part of the application. A real-world e-learning application has plenty of pages and from any page you can add an item to your cart and you see the number of the cart item getting updated.
[00:17 - 00:35] As we know passing data is only possible from parent to child in React and not the other way round. To make our basket data accessible from any page we can either use props which will be quite complicated as data will have to go through the components which don't really need it.
[00:36 - 00:51] We can either keep it to the topmost element such as the app.tsx file and pass the data to the children. We can also use the state management system such as React or Mobix which we are going to do soon.
[00:52 - 01:00] But what if you don't have a huge requirement for centralizing the state? In that case using products can be an overkill.
[01:01 - 01:10] Well, in this situation React context comes to the rescue. It comes with React and does a pretty good job when it comes to centralizing the state.
[01:11 - 01:17] So let's see how to do that. Inside our SAC folder we will need a new folder called context.
[01:18 - 01:39] Inside we can create a new file and we'll call it StowContext.tsx. In simple words we are creating a component which will be a wrapper to our application.
[01:40 - 01:55] Any data or methods made in this component will be shared to the entire application. We'll start with the interface which will have everything we need in our store context.
[01:56 - 02:12] First of all we need a basket which will be of type basket as well. So let me make it store context value.
[02:13 - 02:31] And let's start with the basket which will be of type basket and we can import the model or it can be null. We also need two methods, set basket and remove item.
[02:32 - 02:41] Set basket method will accept a basket as a parameter. So let me write let's call it set basket.
[02:42 - 02:58] And it will accept basket which will be of type basket and it will return void. Remove item method will accept a cause ID and return void.
[02:59 - 03:14] So let's write remove item and it will accept cause ID of type string. And it will return void.
[03:15 - 03:41] Now we will create a method to store our store context. So here let's create export const store context which will be equal to the create context method which we can import from react create context from react.
[03:42 - 04:03] We can give it a type of this store context value store context value or can be undefined because the default value will provide is going to be undefined. We can create a custom hook now and we will call it use store context.
[04:04 - 04:21] So here let's make a function export function use store context. This will return everything we have in our store context.
[04:22 - 04:50] For this we can create a new variable called context context and this will be equal to our store context and we have another hook which we can use that is called use context and it will take the value which is this store context. We can also put a condition which checks if the context is undefined.
[04:51 - 05:01] So let's check if context is undefined. In this case we do not want to return the context.
[05:02 - 05:23] We can throw an error instead. So let's write through error and we can also provide a message which says the store context is currently undefined.
[05:24 - 05:30] Finally if it's not undefined we can return the context. We are not done yet.
[05:31 - 05:33] Now we want to create a store provider. This is going to be a function.
[05:34 - 05:51] So let me write export function store provider. As a parameter all we want to pass are the react components.
[05:52 - 06:17] Since our store context is going to be the wrapper everything inside can be considered as children. So we can simply write children and give it a type props with children which is provided by react and also we need to give it a type.
[06:18 - 06:57] So let's give it type any and now like any react component we will create a state which will store our basket. We can call it basket, const basket and set basket and we will use the use state hook and this can have value of either basket or null.
[06:58 - 07:03] The default value can be null as well. Set basket method is quite straightforward.
[07:04 - 07:11] It just takes the basket and stores it. We can now create remove item function.
[07:12 - 07:30] So let's go down and create function remove item and this will accept cause ID which will be of type string. Now inside we want to check if the basket exists.
[07:31 - 07:47] If not we will simply return. So what I'll do is if no basket return we can then create a copy of our basket items inside const items.
[07:48 - 08:12] So let me write const items and we will use a spread operator inside and we will write basket.dot basket dot items. By doing this we are not mutating the state but creating a new copy of it and make it replace the existing state.
[08:13 - 08:33] Now we can check if the cause ID provided by the client actually exists in our basket. We can find the index of it first and for that we can create a new function and let's call it item index.
[08:34 - 08:58] This will use find index method provided by JavaScript. So what I'll do is write items dot find index and we will pass I which is our item in the basket and check if I dot cause ID is equal to the cause ID.
[08:59 - 09:19] Now if the cause with this ID exists it will give us the index otherwise it will return minus one. Now if the item index is equal to or greater than zero which means the item exists we can use splice method on items which is again a very popular JavaScript method.
[09:20 - 09:25] This helps us to remove the items from the array. So let me write it.
[09:26 - 10:00] First of all I'll check if item index is greater than zero we are checking if the index is there that means the cause exists in the basket. Now we will splice it so we'll use items dot splice and it takes two arguments the first one is the index from where we want to remove it and which is the item index and the second argument is how many items do we want to remove from that particular index and for this we will use just one.
[10:01 - 10:07] We are passing one because we just want to delete one item. Now we want to set the new items to be in the basket.
[10:08 - 10:37] So what I'll do is use set basket and I can use previous state so I can use priv state and can inside I will return dot dot dot brief state and now the new items. We just want to say that it is not null so we will pass an exclamation.
[10:38 - 10:59] We are using this because this is just an additional check to keep our items intact. Finally we want to return everything we can either use store context dot provider or store context dot consumer since we are providing the data we will use provider.
[11:00 - 11:29] So here I will return and we will use store context dot provider. We are getting the error because we are not providing the value and as values we want to use the basket, set basket and remove item and inside I will simply use children.
[11:30 - 11:45] And now we are going to wrap our store context so that every child has access to all its values and methods to the highest level in our application which is the index dot tss file. So let's go there we will use index dot tss.
[11:46 - 12:05] So we can wrap our app component with the store provider. So here we can use store provider and let me copy it and paste it here.
[12:06 - 12:15] By doing this everyone in our app component will have access to our store values and the methods. So let's see how to consume the methods in the next lesson.