Using Types
In this lesson, we're going to learn how to use types in TypeScript
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
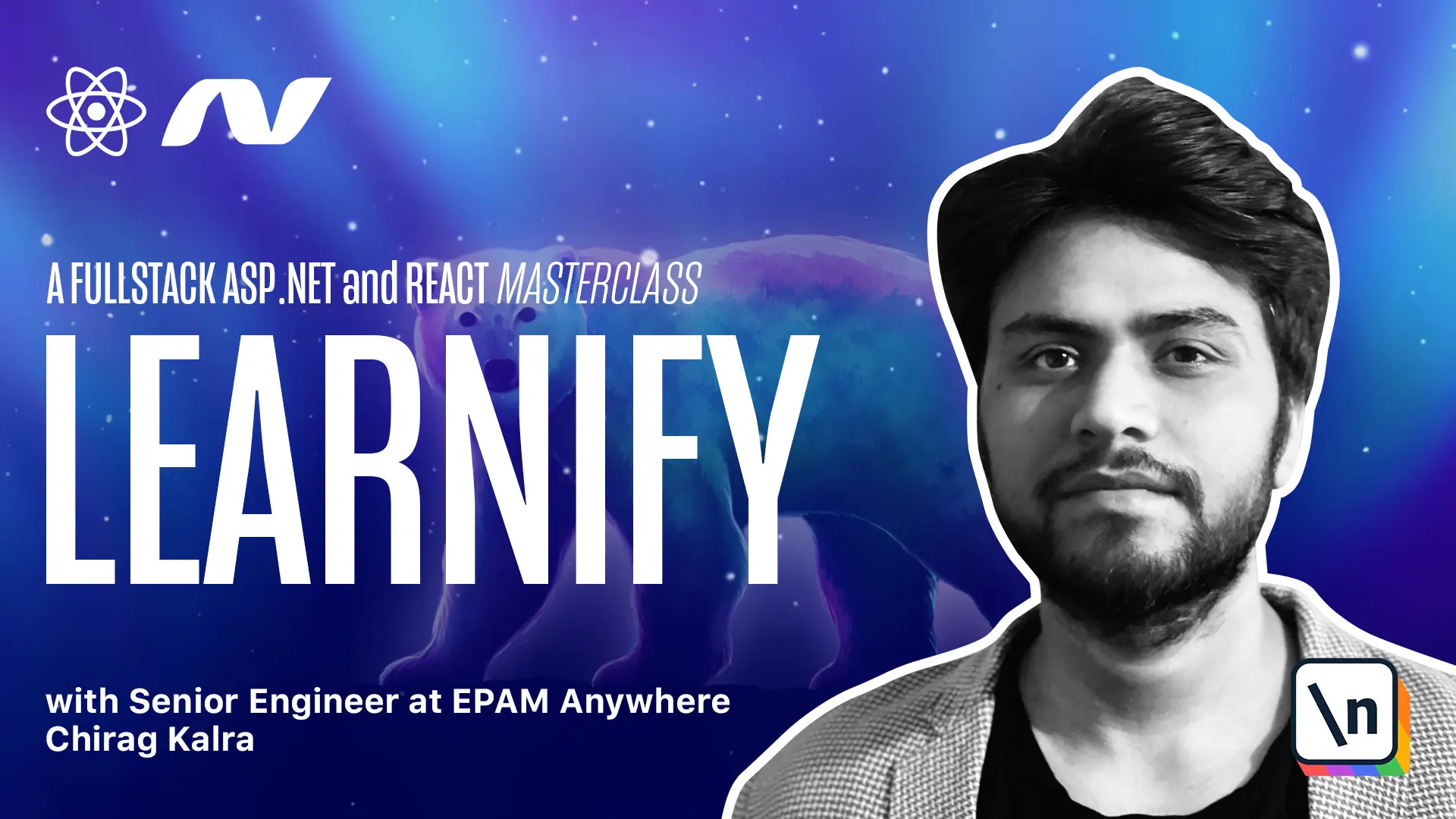
[00:00 - 00:09] I have talked briefly about types in TypeScript in the second module of this course. In this lesson, we will use them practically.
[00:10 - 00:19] Open the project and run npm start. We see nothing because there is no app.js file yet.
[00:20 - 00:30] Let's write our code inside app.ts and later we will compile it back to JavaScript. We know that JavaScript has three primitive data types.
[00:31 - 00:36] Number, string and Boolean. JavaScript doesn't have float or decimal data type.
[00:37 - 00:49] All of these come under number data type. Let's create a const num and give it a value 1.
[00:50 - 01:10] And another const called num2 and give it a value 2. Let's create a string constant str and give it a value iamstring.
[01:11 - 01:26] And finally, a Boolean bool and let's give it a value true. TypeScript has a property called inference which is the ability to infer the data type.
[01:27 - 01:36] Now that we have assigned values to our constants, there is no need to give them a type explicitly. Although we can do that but it will be overkill to do so.
[01:37 - 01:49] But let's say if it were let rather than const and we needed to change the value. Let's change const to let.
[01:50 - 02:08] Now if we hover over num it has inferred the data type number. If we try to give it a value of a string it gives us an error and it says type string is not assignable to type number.
[02:09 - 02:18] It is happening because we are inside a typeScript file. If it were a JavaScript file there would have been no issues assigning another data type to the variable.
[02:19 - 02:30] Now let's change the string to let and try to change the data type to a number. Again, it gives us an error.
[02:31 - 02:38] I hope you understand what inference means. Let's change it back to const.
[02:39 - 02:55] Now let's create a function and let's call it do something. As parameters let's put arg1 and arg2.
[02:56 - 03:09] We can do anything we want to with it. Let's console log arg1 plus arg2 and let's call this function.
[03:10 - 03:20] Now we can pass any parameters here since we haven't told typeScript what we are expecting. We can even pass boolean and it won't complain.
[03:21 - 03:29] This is why giving a type is important while passing parameters to function. If now we give the parameters type number.
[03:30 - 03:51] See now it complains but if I change arg2 to a boolean and the first one to a number. Now the console gives us the error because we can't use the operator with a boolean.
[03:52 - 03:57] Isn't it cool? TypeScript is telling us what we are doing is wrong before even we ran the code .
[03:58 - 04:13] If I change boolean to a string and pass a string here. It won't complain because you can concatenate a string and a number.
[04:14 - 04:26] I hope you understand the purpose of the video. In short it's a good idea to pass types to parameters of the function but not to the variables because typeScript can easily infer it.
[04:27 - 04:33] There can be a use case where you are just declaring a variable. Let's call it def.
[04:34 - 04:47] In this case it's a good idea to tell typeScript what you are expecting. If I give it a type number and try to assign it to a string it will give us the error.
[04:48 - 05:02] This is what inference does to your code. Let's change arg2 back to a number and pass num and num2 to a function.
[05:03 - 05:21] To run our code we will have to compile it to JavaScript. I hope your server is running so open another terminal and type tseapp.ts.
[05:22 - 05:28] It will create a compiled JavaScript file. If we see it there is no typeScript here.
[05:29 - 05:40] Another good thing about light server is that you don't have to stop and restart the server again and again. Open the browser and open the console.
[05:41 - 05:44] See there is the value 3 from our function. Amazing.
[05:45 - 05:49] I hope you understand how inference works in TypeScript and I will see you in the next lesson.