Adding Eager Loading
In this lesson, we're going to add code for eager loading
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React with a single-time purchase.
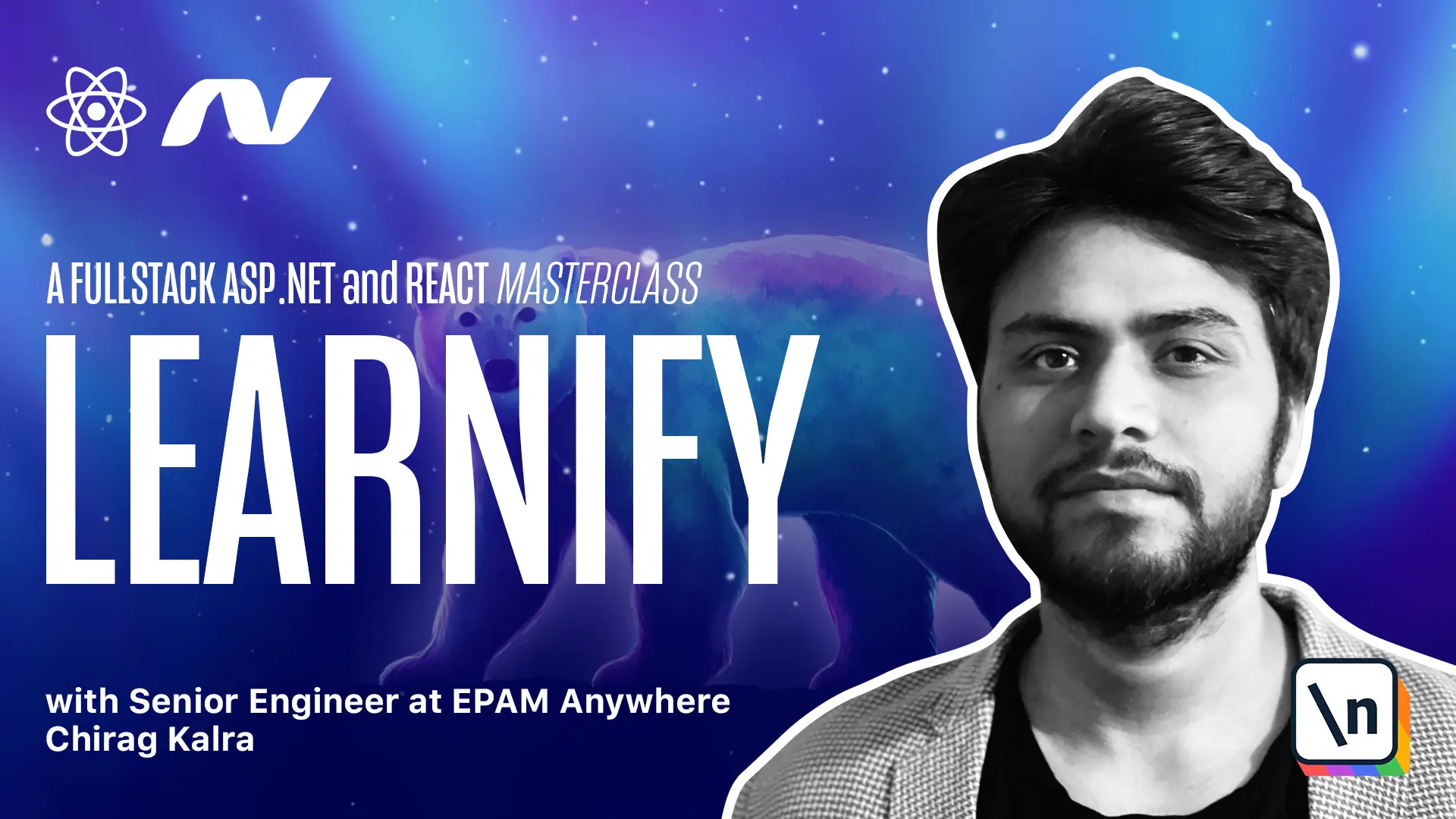
[00:00 - 00:06] We saw that our requests are not returning data from another table. Category request doesn't show courses.
[00:07 - 00:13] Courses request does not show category, learnings and requirements. This is what we want to fix right now.
[00:14 - 00:31] This is called loading of navigation properties. For this, we can either use lazy loading, which means entity framework will add all the navigation properties to our response every single time, which is not ideal because you don't have a choice of what to return and what not to.
[00:32 - 00:44] We have a better option of using eager loading, where we are explicitly telling entity framework, which navigation property to load alongside the returning entity. This is a context feature.
[00:45 - 00:51] So we'll have to go to the implementation of our interfaces. So the category repository and course repository.
[00:52 - 01:13] Inside get categories async method, before to list async, we need to append our include request, because to list async is the call to the database, and we need to do it before. Using include statement will ask the database for navigation property that we want in our response.
[01:14 - 01:19] We want to include courses in the get categories. So we can use lambda expression.
[01:20 - 01:27] Let's make it down. And here we will write include.
[01:28 - 01:38] And here we need a lambda statement, which will say we want c.courses. And that's it.
[01:39 - 01:55] We need to do the same thing for the get category by ID async method. Again, we will bring it down and .include c, which goes to c.courses.
[01:56 - 02:10] We will receive an error because find async method doesn't accept i queryable. This is i queryable because we are building a query before using to list async or find async.
[02:11 - 02:18] If we don't have the include statement, it's not i queryable. But now by adding this include statement, it is.
[02:19 - 02:26] So we'll have to replace it with first or default async. And that will again take a lambda expressions.
[02:27 - 02:39] We can write x, which goes to x.id, which is equal to id. Now we have to do the same thing inside our course repository.
[02:40 - 02:53] Let's go there. And let's include c, which goes to c.categories.
[02:54 - 03:15] And again, we'll have to change find async to first or default async here. And again, inside we need a lambda expression, x goes to x.id, which is equal to id.
[03:16 - 03:30] And finally, we need to do this here as well. .include c.c.category.
[03:31 - 03:44] Apart from this, we can also add our learnings and requirements table. So include c, which goes to c.learnings.
[03:45 - 04:02] And the same way include c, which goes to c.requirements. I am going to add it only to the get course by ID async method, because we are not going to use it when we are calling all the courses.
[04:03 - 04:12] We will call them just when we want to show the main information about those courses. Learnings and details will be important in the description page.
[04:13 - 04:21] But I can call the category with this request. So I have included the category in the get courses async method.
[04:22 - 04:30] Now if I open postman, let's see what happens. Let me try to make get courses request.
[04:31 - 04:42] I have system.text.json exception. This says a possible object cycle was detected.
[04:43 - 04:46] You know what's wrong here? Okay, let me show it to you.
[04:47 - 04:58] Our course has category and our category has collection of course. So this is making a circular dependency with each other.
[04:59 - 05:06] Now when we are calling a course, it is calling a category. Category again is calling a course, and the cycle repeats itself.
[05:07 - 05:10] So how to resolve this issue? Let's look at that in the next lesson.