Getting Payment Intent from the Server
In this lesson, we're going to make an API call to get payment intent from the server
Get the project source code below, and follow along with the lesson material.
Download Project Source CodeTo set up the project on your local machine, please follow the directions provided in the README.md
file. If you run into any issues with running the project source code, then feel free to reach out to the author in the course's Discord channel.
Lesson Transcript
[00:00 - 00:08] Now that we know how our checkout page looks like, let's start preparing the payment process. So first of all, let's close everything.
[00:09 - 00:19] And to make the payment from our front end, we need payment intent ID and decline secret, which we will receive from our server. Before that, let's make some changes to our basket model.
[00:20 - 00:28] So let's search for basket and models. Inside the basket, we will add payment intent ID.
[00:29 - 00:48] So let's write payment intent ID, which will have a type string and client secret of type string as well. Both of them are optional and can be null.
[00:49 - 00:59] So we can add a question mark on both of these properties. And now let's go to the agent file and add another endpoint for payments.
[01:00 - 01:07] So agent and below the baskets, we can create another endpoint. Let's call it payments.
[01:08 - 01:12] We only have one endpoint. So we can call it payment intent.
[01:13 - 01:21] So let me write payment intent. We are going to make a post request with the endpoint being payments.
[01:22 - 01:29] So let's write requests dot post. And the endpoint is payments.
[01:30 - 01:39] So we can write payments. And since it's a post request and we are not passing any body, so we can use an empty object.
[01:40 - 01:45] And from this request, we will receive basket as a response. So we can write basket.
[01:46 - 01:51] Now finally, we can export it. And it should work.
[01:52 - 02:06] Now we need to make this request when the client is ready to make the payment. That happens when the client clicks on checkout, which means inside the checkout page, whenever the component first renders, which means inside the checkout page.
[02:07 - 02:12] So let's go to the checkout page. So we will make this request whenever this component first renders.
[02:13 - 02:18] And for that, we will use a use effect hook. So here let's use use effect.
[02:19 - 02:32] And before writing the API call, let's see if it is as expected. And yeah, we forgot to add it as a function.
[02:33 - 02:45] Now we can go back to the function and inside the user effect hook, we could call agent dot payments dot payment intent. And from this API call, we will receive a basket.
[02:46 - 02:55] So let me write basket. And this time the basket will have a payment intent ID and a client secret.
[02:56 - 03:10] So what we can do is we can dispatch the set basket function so that the basket in our Redux state can get the payment intent ID and the client secret. So here, let me write dispatch.
[03:11 - 03:25] And we will use the use app dispatch. And now when we receive this basket, we can dispatch the set basket, reduce the function and we can pass the basket.
[03:26 - 03:30] We can also catch some errors. So let me write dot catch.
[03:31 - 03:45] And if there is any error, we can simply log it for now. And let's also mention the dependency of dispatch.
[03:46 - 03:55] And as you know, this request is an authorized request. So we won't be able to make this request until we have an authorization token inside the request header.
[03:56 - 04:17] So what we can do is we can go to the agent dot PS file once again and make a function, which will be called XOs interceptor. So let's go on top and here, let's create a function which will be called XOs interceptor.
[04:18 - 04:27] This function will check if we have a token inside our Redux store. And if there is, it will attach the token inside the request on the authorization header.
[04:28 - 04:46] So we need to pass store as a parameter, which will have a type store, which we will import from Redux. Now inside, we can write XOs dot interceptors dot request dot use.
[04:47 - 04:57] And inside, we will get a config, which is basically a configuration. And inside the callback function, we can check if we have the token.
[04:58 - 05:07] So we can write const token is equal to, we will use the store. And from the store, we will use get state.
[05:08 - 05:24] And then the user dot user dot token, because this is where our token is located. And we can use a question mark because the user can be null.
[05:25 - 05:48] Now we will check if we have a token, in that case, we will use config dot headers dot authorization. That will be equal to a bearer and inside, we will pass the token.
[05:49 - 06:02] And finally, from this function, we will return the config. We are making this a function because if we try to get access of the store here in this file, it might give us some errors.
[06:03 - 06:14] So we can export it from here. After exporting it, we can call this function inside the index dot tss file.
[06:15 - 06:24] So let's go to the index dot tss file. And on top, we can use the xio's interceptor function.
[06:25 - 06:33] And we will pass the store, which will be imported from our configure store. Now let's check the terminal if everything is working fine.
[06:34 - 06:49] And now we can open the browser to check what we have inside basket. First of all, let's clear the basket and go to the home page and check what do we have inside our basket.
[06:50 - 07:02] As you can see, our payment intent ID and declined secret is null. Now let's add something to the cart and let's click on checkout.
[07:03 - 07:15] Now if we open that again and here, let's check what is inside the basket. We also have a payment intent ID and a client secret, which means our implementation is working fine.
[07:16 - 07:19] Now in the next lecture, let's use these and try to make the payment.
This lesson preview is part of the The newline Guide to Fullstack ASP.NET Core and React course and can be unlocked immediately with a \newline Pro subscription or a single-time purchase. Already have access to this course? Log in here.
Get unlimited access to The newline Guide to Fullstack ASP.NET Core and React, plus 70+ \newline books, guides and courses with the \newline Pro subscription.
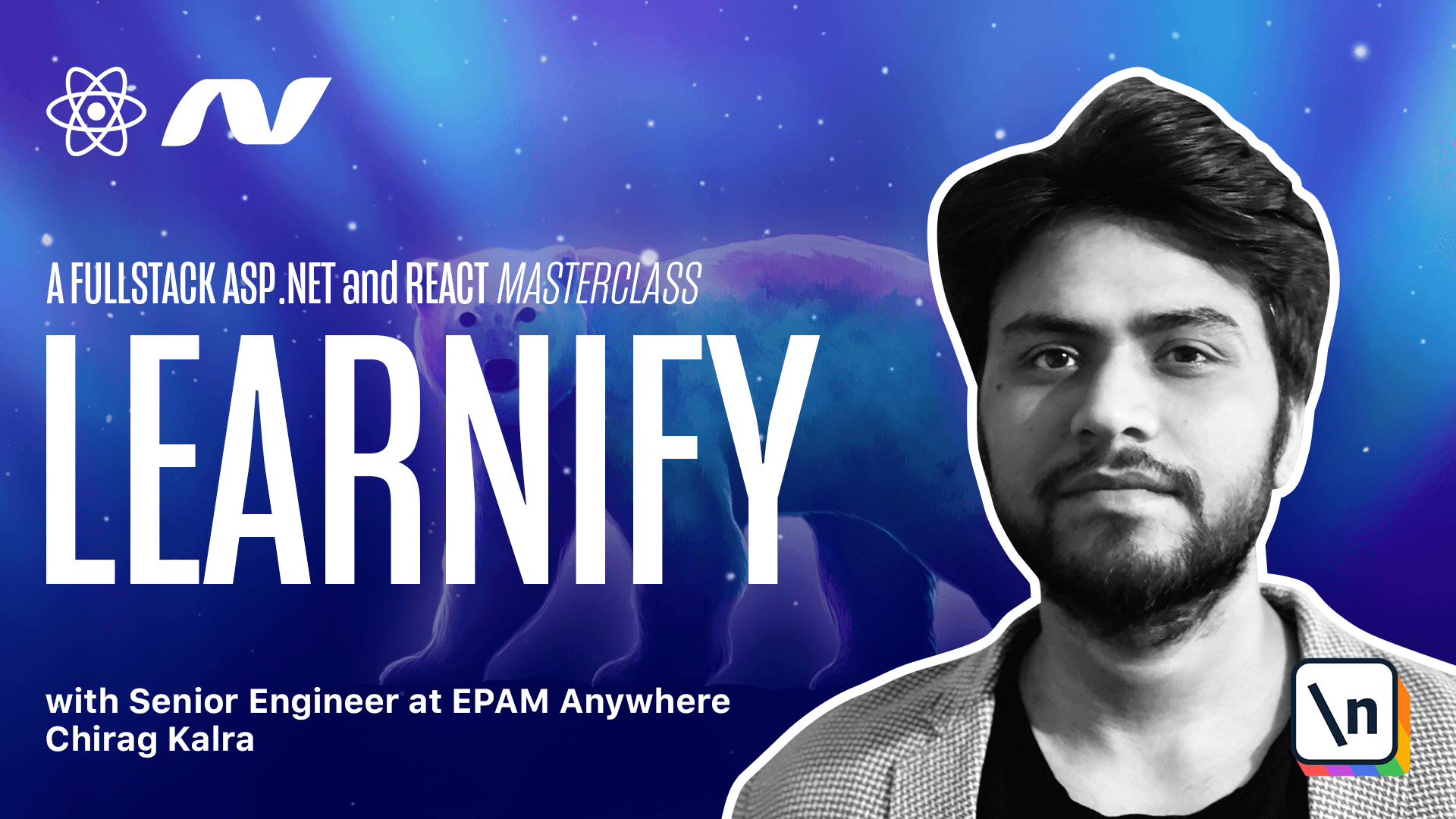